Transfer via transition account
Paysera offers a secure API that lets you collect money and then distribute it using a transition account. This allows making one payment from various payers, also split one received payment to various number of receivers.
Allowance creation diagram
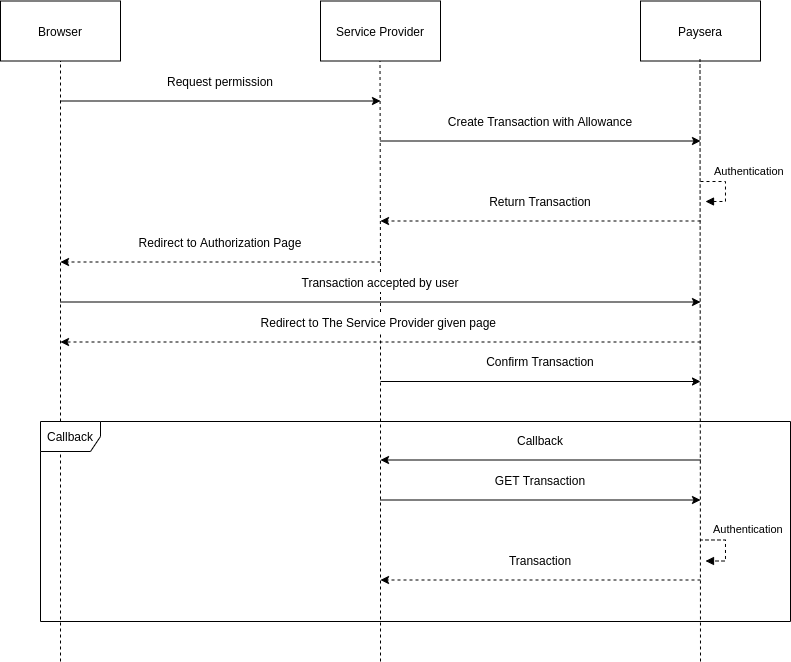
Transfer via transition account diagram
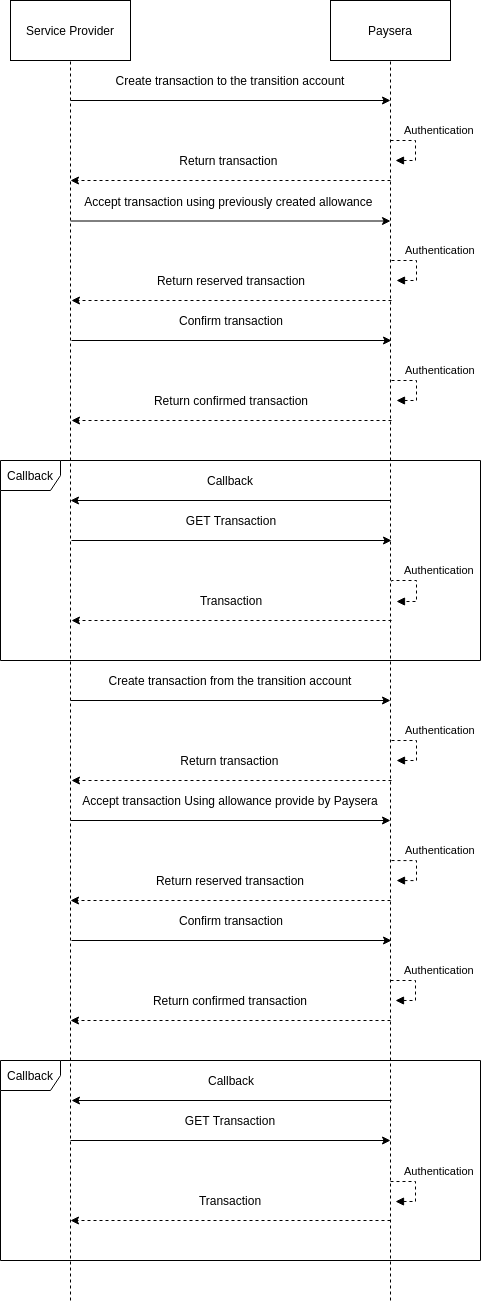
Integration
1. Create an allowance for background payment
Create an allowance using the library
Allowance is a permission to make payments without asking the user permission for each payment. It allows to make small payments from wallet very quickly and smoothly, after s/he has confirmed client-initiated allowance or created one by himself/herself.
1. Create a folder.
Create a folder, name it paysera-wallet. Here, all the files related to payment creation will be stored.
2. Download the library.
Download GitHub files to the paysera-wallet folder.
3. Create a file that will create an allowance.
Create file allowance.php in the paysera-wallet folder. First of all you must include library and
register GatewayClient_Autoloader
as an SPL autoloader:
<?php if (!class_exists('Paysera_WalletApi_Autoloader')) { require_once 'lib-wallet-php-client-master/src/Paysera/WalletApi/Autoloader.php'; } Paysera_WalletApi_Autoloader::register();
Write in your authentication data values, which you have received as Wallet credentials:
// $clientId - mac $clientId = 'wkVd93h2uS'; // $secret - mac_key $secret = 'IrdTc8uQodU7PRpLzzLTW6wqZAO6tAMU';
Create main object to use for all functionality. Using our library you may create object either for production, either for testing – Sandbox:
Production$api = new Paysera_WalletApi($clientId, $secret);Sandbox
$api = new Paysera_WalletApi($clientId, $secret, Paysera_WalletApi_Util_Router::createForSandbox());
Start new session as we will store transactionKey in $_SESSION['transactionKey']
:
session_start();
To facilitate the catching of potential exceptions code must be surrounded in try
block:
try { // Allowance creation steps } catch (Exception $e) { echo '<pre>', $e, '</pre>'; }
Inside try block there will be logic which creates and confirms allowance. First step is to create an allowance object, then create a transaction with a created allowance object, then authorise transaction and the last step - client (developed system) must confirm that transaction. Transaction might be confirmed automatically, after redirect to client (developed system) site or after callback. See Transaction resource for more information.
To create allowance object we need to create price
which is used in allowance object:
$price = Paysera_WalletApi_Entity_Money::create() ->setAmountInCents(500) ->setCurrency('EUR') ;
Create an allowance object. In this example we create allowance with max price and make it valid for a week.
More info about all parameters: Allowance resource.
In order to create allowance object and add parameters to it we use
Paysera_WalletApi_Entity_Payment
:
$allowance = Paysera_WalletApi_Entity_Allowance::create() ->setDescription('Allowance of 5.00 EURO for 7 days') ->setMaxPrice($price) ->setValidFor(604800) ;
Transaction object is created and values are updated using Paysera_WalletApi_Entity_Transaction
.
In this example we are adding only allowance with a redirect link which will be used after user confirms
transaction:
$transaction = Paysera_WalletApi_Entity_Transaction::create() ->addPayment($payment) ->setRedirectUri('http://wallet.dev.docker/allowance.php') ;
After we get transaction object we are creating the transaction:
$transactionCreated = $api->walletClient()->createTransaction($transaction);
User is redirected to the transaction confirmation page with a code:
header('Location:' . $api->router()->getTransactionConfirmationUri($transactionCreated->getKey()));
After user accepts transaction client (developed system) must confirm it:
$transaction = $api->walletClient()->getTransaction($_SESSION['transactionKey']) $api->walletClient()->confirmTransaction($transaction->getKey());
The whole code in the file allowance.php:
<?php if (!class_exists('Paysera_WalletApi_Autoloader')) { require_once 'lib-wallet-php-client-master/src/Paysera/WalletApi/Autoloader.php'; } Paysera_WalletApi_Autoloader::register(); // $clientId - mac $clientId = 'wkVd93h2uS'; // $secret - mac_key $secret = 'IrdTc8uQodU7PRpLzzLTW6wqZAO6tAMU'; $api = new Paysera_WalletApi($clientId, $secret, Paysera_WalletApi_Util_Router::createForSandbox()); session_start(); try { if (!isset($_SESSION['transactionKey'])) { $price = Paysera_WalletApi_Entity_Money::create() ->setAmountInCents(500) ->setCurrency('EUR') ; $allowance = Paysera_WalletApi_Entity_Allowance::create() ->setDescription('Allowance of 5.00 EURO for 7 days') ->setMaxPrice($price) ->setValidFor(604800) ; $transaction = Paysera_WalletApi_Entity_Transaction::create() ->setAllowance($allowance) ->setRedirectUri('http://wallet.dev.docker/allowance.php') ; $transactionCreated = $api->walletClient()->createTransaction($transaction); $_SESSION['transactionKey'] = $transactionCreated->getKey(); header('Location:' . $api->router()->getTransactionConfirmationUri($transactionCreated->getKey())); } if (isset($_SESSION['transactionKey'])){ $transaction = $api->walletClient()->getTransaction($_SESSION['transactionKey']); // ToDo: some action with $transaction if ($transaction->getStatus() === 'reserved') { $api->walletClient()->confirmTransaction($transaction->getKey()); } unset($_SESSION['transactionKey']); } } catch (Exception $e) { echo '<pre>', $e, '</pre>'; }
Create an allowance using the specifications
Allowance is a permission to make payments without asking the user permission for each payment. It allows to make small payments from wallet very quickly and smoothly, after s/he has confirmed client-initiated allowance or created one by himself/herself.
This method creates allowance in Paysera system. After creating allowance, user should be redirected to confirmation page or allowance should be confirmed in other ways. More info about allowance: Allowance Resource.
new
are deleted automatically 1 month after creation time.POST https://wallet.paysera.com/rest/v1/allowance
Example request for allowance without limits
POST /rest/v1/allowance HTTP/1.1 Host: wallet.paysera.com Content-Type: application/json;charset=utf-8 User-Agent: Paysera WalletApi PHP library Authorization: MAC id="wkVd93h2uS", ts="1343811600", nonce="nQnNaSNyubfPErjRO55yaaEYo9YZfKHN", mac="qHnDddnmudMi6FwQeWeINW2zEGNJR6xFrbpWOdgggSI=", ext="body_hash=Y2nyrCCNhAqRbJu0UK8b57S%2BDim5jcyCsRQoz9My4j0%3D"
{ "description": "Allowance for weekly services (5 weeks)", "currency": "EUR", "max_price": 1500, "valid": { "for": 3110400 } }
Example response
HTTP/1.1 200 OK Content-type: application/json;charset=utf-8
{ "id": 2987, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "new", "description": "Allowance for weekly services (5 weeks)", "currency": "EUR", "max_price": 1500, "max_price_decimal": "15.00" }
2. Perform background payment to the transition account with previously created allowance
Background payment with a library
Create a file that will make background payment.
Create file pay-with-allowance.php in the paysera-wallet folder. the process is similar to Create a payment, just difference is that client (developed system) doesn't need to wait for user confirmation as created allowance will be added to transaction. The sample code which makes background payment with allowance:
<?php if (!class_exists('Paysera_WalletApi_Autoloader')) { require_once 'lib-wallet-php-client-master/src/Paysera/WalletApi/Autoloader.php'; } Paysera_WalletApi_Autoloader::register(); // $clientId - mac $clientId = 'wkVd93h2uS'; // $secret - mac_key $secret = 'IrdTc8uQodU7PRpLzzLTW6wqZAO6tAMU'; $api = new Paysera_WalletApi($clientId, $secret, Paysera_WalletApi_Util_Router::createForSandbox()); try { $price = Paysera_WalletApi_Entity_Money::create() ->setAmountInCents(100) ->setCurrency('EUR') ; $item = Paysera_WalletApi_Entity_Item::create() ->setTitle('Item') ->setDescription('Item in sale') ->setImageUri('https://developers.paysera.com/bundles/evpfrontpage/img/logo.png') ->setPrice($price) ->setQuantity(2) ; $fullPrice = Paysera_WalletApi_Entity_Money::create() ->setAmountInCents(200) ->setCurrency('EUR') ; $payment = Paysera_WalletApi_Entity_Payment::create() ->addItem($item) ->setPrice($fullPrice) ->setDescription('In Sale') ; // if commission is needed $commissionPrice = Paysera_WalletApi_Entity_Money::create() ->setAmountInCents(100) ->setCurrency('EUR') ; $commission = Paysera_WalletApi_Entity_Commission::create() ->setOutCommission($commissionPrice) ; $payment = $payment ->setCommission($commission) ; $allowanceTransaction = $api->walletClient()->getTransaction('[ALLOWANCE_TRANSACTION_KEY]'); $transaction = Paysera_WalletApi_Entity_Transaction::create() ->addPayment($payment) ->setAllowance($allowanceTransaction->getAllowance()) ; $transactionCreated = $api->walletClient()->createTransaction($transaction); $api->walletClient()->acceptTransactionUsingAllowance( $transactionCreated->getKey(), $allowanceTransaction->getWallet() ); // ToDo: some actions with transaction $api->walletClient()->confirmTransaction($transactionCreated->getKey()); } catch (Exception $e) { echo '<pre>', $e, '<pre>'; }
Background payment with the specification
1. Create transaction.
Transaction groups one or more objects into one confirmable group. To confirm any created object, there must be a transaction.
Moreover, transactions take part in all-or-nothing scenarios: either all of grouped payments are done
successfully, or all fail. For example, if wallet has sufficient account balance only for one of grouped
payments, user will be unable to confirm this transaction.
Transactions are also good when making shop
carts with payments to different beneficiaries - user will have to confirm something just once.
This method creates transaction that groups payment(s) and/or allowance into item that can be confirmed. More info about transaction creation: Transaction resource.
POST https://wallet.paysera.com/rest/v1/transaction
Example request for creating payment and assigning optional allowance
POST /rest/v1/transaction HTTP/1.1 Host: wallet.paysera.com Content-Type: application/json;charset=utf-8 User-Agent: Paysera WalletApi PHP library Authorization: MAC id="wkVd93h2uS", ts="1343811600", nonce="nQnNaSNyubfPErjRO55yaaEYo9YZfKHN", mac="R5Kw+qeaej/TRdVEkxbKzJHeHfA7HbsEcI4StcJo3lU=", ext="body_hash=gK8kVbYW1XEeZUf4BR1yZ45YLu%2BEYnq1WOGYtRhxyQA%3D"
{ "payments": [ { "description": "Payment for order No. 1234", "price": 1299, "currency": "EUR", "parameters": { "orderid": 1234 } } ], "allowance": { "id": 784, "optional": true }, "redirect_uri": "http://www.example.com/somePage" }
Example response
HTTP/1.1 200 OK Content-type: application/json;charset=utf-8
{ "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "new", "project_id": 2248, "valid_for_payment_card_debit": false, "payments": [ { "id": 2988, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "new", "price": 1299, "currency": "EUR", "price_decimal": "12.99", "description": "Payment for order No. 1234", "parameters": { "orderid": 1234 } } ], "allowance": { "optional": true, "data": { "id": 784, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "new", "description": "Allowance for weekly services (5 weeks)", "currency": "EUR", "max_price": 1500, "max_price_decimal": "15.00", "limits": [ { "max_price": 300, "max_price_decimal": "3.00", "time": 604800 } ] } }, "reserve": { "until": 1355400732 }, "use_allowance": false, "suggest_allowance": false, "auto_confirm": false, "redirect_uri": "http://www.example.com/somePage" }
2. Authorise transaction.
After creating transaction, it has to be authorised by a user and confirmed by the client (developed system) to take effect. Authorising (reserving funds) can be accomplished using active allowance, without user intervention. This allowance must be accepted beforehand in some other way and confirmed by the client (developed system).
More info about authorising transaction: Authorising transactions (reserving funds).
Transaction cannot have an included allowance. Allowance must be accepted beforehand in some other way and confirmed by the client (developed system). More info about allowance: Allowance resource.
PUT https://wallet.paysera.com/rest/v1/transaction/:transaction_key/reserve/:wallet
Example request
PUT /rest/v1/transaction/pDAlAZ3z/reserve/14471 HTTP/1.1 Host: wallet.paysera.com User-Agent: Paysera WalletApi PHP library Authorization: MAC id="wkVd93h2uS", ts="1343811600", nonce="nQnNaSNyubfPErjRO55yaaEYo9YZfKHN", mac="hKC//dWjcCRuPwlXVlDyo6tdzlRbRy/CCNLRfbvzvDw="
transaction_key
is
pDAlAZ3z
in the wallet which ID is 14471
.Example response
HTTP/1.1 200 OK Content-type: application/json;charset=utf-8
{ "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "reserved", "type": "automatic", "wallet": 14471, "valid_for_payment_card_debit": false, "project_id": 2248, "payments": [ { "id": 2988, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "reserved", "price": 1299, "currency": "EUR", "price_decimal": "12.99", "wallet": 14471, "freeze": { "until": 1357992732 }, "description": "Payment for order No. 1234", "parameters": { "orderid": 1234 }, "transfer_id": 578842 } ], "reserve": { "until": 1357992732 }, "use_allowance": false, "suggest_allowance": false, "auto_confirm": false }
3. Confirm transaction.
When transaction status is reserved, you can confirm or revoke the transaction.
PUT https://wallet.paysera.com/rest/v1/transaction/:transaction_key/confirm
Confirmed transaction is returned on success. See get transaction information response data structure for more information.
Example request
PUT /rest/v1/transaction/pDAlAZ3z/confirm HTTP/1.1 Host: wallet.paysera.com User-Agent: Paysera WalletApi PHP library Authorization: MAC id="wkVd93h2uS", ts="1343811600", nonce="nQnNaSNyubfPErjRO55yaaEYo9YZfKHN", mac="ZQYIS0L4Uq40te+qxNXJzWHNO6Ff7qhnEDuwduFlqRI="
Example response
HTTP/1.1 200 OK Content-type: application/json;charset=utf-8
{ "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "confirmed", "type": "page", "wallet": 14471, "valid_for_payment_card_debit": false, "confirmed_at": 1355314392, "project_id": 2248, "payments": [ { "id": 2988, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "confirmed", "price": 1299, "currency": "EUR", "price_decimal": "12.99", "wallet": 14471, "confirmed_at": 1355314392, "freeze": { "until": 1357992732 }, "description": "Payment for order No. 1234", "parameters": { "orderid": 1234 } } ], "allowance": { "optional": true, "data": { "id": 784, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "active", "currency": "EUR", "wallet": 14471, "confirmed_at": 1355314392, "valid": { "until": 1357992732 }, "description": "Allowance for weekly services (5 weeks)", "max_price": 1500, "max_price_decimal": "15.00", "limits": [ { "max_price": 300, "max_price_decimal": "3.00", "time": 604800 } ] } } }
3. Perform background payment from the transition account
Transfer from transition account is the same as the transfer from regular account. The difference is that transition account is owned by Paysera and you cannot store money in it.
Background payment with a library
Create a file that will make background payment.
Create file pay-with-allowance.php in the paysera-wallet folder. the process is similar to Create a payment, just difference is that client (developed system) doesn't need to wait for user confirmation as created allowance will be added to transaction. The sample code which makes background payment with allowance:
<?php if (!class_exists('Paysera_WalletApi_Autoloader')) { require_once 'lib-wallet-php-client-master/src/Paysera/WalletApi/Autoloader.php'; } Paysera_WalletApi_Autoloader::register(); // $clientId - mac $clientId = 'wkVd93h2uS'; // $secret - mac_key $secret = 'IrdTc8uQodU7PRpLzzLTW6wqZAO6tAMU'; $api = new Paysera_WalletApi($clientId, $secret, Paysera_WalletApi_Util_Router::createForSandbox()); try { $price = Paysera_WalletApi_Entity_Money::create() ->setAmountInCents(100) ->setCurrency('EUR') ; $item = Paysera_WalletApi_Entity_Item::create() ->setTitle('Item') ->setDescription('Item in sale') ->setImageUri('https://developers.paysera.com/bundles/evpfrontpage/img/logo.png') ->setPrice($price) ->setQuantity(2) ; $fullPrice = Paysera_WalletApi_Entity_Money::create() ->setAmountInCents(200) ->setCurrency('EUR') ; $payment = Paysera_WalletApi_Entity_Payment::create() ->addItem($item) ->setPrice($fullPrice) ->setDescription('In Sale') ; // if commission is needed $commissionPrice = Paysera_WalletApi_Entity_Money::create() ->setAmountInCents(100) ->setCurrency('EUR') ; $commission = Paysera_WalletApi_Entity_Commission::create() ->setOutCommission($commissionPrice) ; $payment = $payment ->setCommission($commission) ; $allowanceTransaction = $api->walletClient()->getTransaction('[ALLOWANCE_TRANSACTION_KEY]'); $transaction = Paysera_WalletApi_Entity_Transaction::create() ->addPayment($payment) ->setAllowance($allowanceTransaction->getAllowance()) ; $transactionCreated = $api->walletClient()->createTransaction($transaction); $api->walletClient()->acceptTransactionUsingAllowance( $transactionCreated->getKey(), $allowanceTransaction->getWallet() ); // ToDo: some actions with transaction $api->walletClient()->confirmTransaction($transactionCreated->getKey()); } catch (Exception $e) { echo '<pre>', $e, '<pre>'; }
Background payment with the specification
1. Create transaction.
Transaction groups one or more objects into one confirmable group. To confirm any created object, there must be a transaction.
Moreover, transactions take part in all-or-nothing scenarios: either all of grouped payments are done
successfully, or all fail. For example, if wallet has sufficient account balance only for one of grouped
payments, user will be unable to confirm this transaction.
Transactions are also good when making shop
carts with payments to different beneficiaries - user will have to confirm something just once.
This method creates transaction that groups payment(s) and/or allowance into item that can be confirmed. More info about transaction creation: Transaction resource.
POST https://wallet.paysera.com/rest/v1/transaction
Example request for creating payment and assigning optional allowance
POST /rest/v1/transaction HTTP/1.1 Host: wallet.paysera.com Content-Type: application/json;charset=utf-8 User-Agent: Paysera WalletApi PHP library Authorization: MAC id="wkVd93h2uS", ts="1343811600", nonce="nQnNaSNyubfPErjRO55yaaEYo9YZfKHN", mac="R5Kw+qeaej/TRdVEkxbKzJHeHfA7HbsEcI4StcJo3lU=", ext="body_hash=gK8kVbYW1XEeZUf4BR1yZ45YLu%2BEYnq1WOGYtRhxyQA%3D"
{ "payments": [ { "description": "Payment for order No. 1234", "price": 1299, "currency": "EUR", "parameters": { "orderid": 1234 } } ], "allowance": { "id": 784, "optional": true }, "redirect_uri": "http://www.example.com/somePage" }
Example response
HTTP/1.1 200 OK Content-type: application/json;charset=utf-8
{ "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "new", "project_id": 2248, "valid_for_payment_card_debit": false, "payments": [ { "id": 2988, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "new", "price": 1299, "currency": "EUR", "price_decimal": "12.99", "description": "Payment for order No. 1234", "parameters": { "orderid": 1234 } } ], "allowance": { "optional": true, "data": { "id": 784, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "new", "description": "Allowance for weekly services (5 weeks)", "currency": "EUR", "max_price": 1500, "max_price_decimal": "15.00", "limits": [ { "max_price": 300, "max_price_decimal": "3.00", "time": 604800 } ] } }, "reserve": { "until": 1355400732 }, "use_allowance": false, "suggest_allowance": false, "auto_confirm": false, "redirect_uri": "http://www.example.com/somePage" }
2. Authorise transaction.
After creating transaction, it has to be authorised by a user and confirmed by the client (developed system) to take effect. Authorising (reserving funds) can be accomplished using active allowance, without user intervention. This allowance must be accepted beforehand in some other way and confirmed by the client (developed system).
More info about authorising transaction: Authorising transactions (reserving funds).
Transaction cannot have an included allowance. Allowance must be accepted beforehand in some other way and confirmed by the client (developed system). More info about allowance: Allowance resource.
PUT https://wallet.paysera.com/rest/v1/transaction/:transaction_key/reserve/:wallet
Example request
PUT /rest/v1/transaction/pDAlAZ3z/reserve/14471 HTTP/1.1 Host: wallet.paysera.com User-Agent: Paysera WalletApi PHP library Authorization: MAC id="wkVd93h2uS", ts="1343811600", nonce="nQnNaSNyubfPErjRO55yaaEYo9YZfKHN", mac="hKC//dWjcCRuPwlXVlDyo6tdzlRbRy/CCNLRfbvzvDw="
transaction_key
is
pDAlAZ3z
in the wallet which ID is 14471
.Example response
HTTP/1.1 200 OK Content-type: application/json;charset=utf-8
{ "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "reserved", "type": "automatic", "wallet": 14471, "valid_for_payment_card_debit": false, "project_id": 2248, "payments": [ { "id": 2988, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "reserved", "price": 1299, "currency": "EUR", "price_decimal": "12.99", "wallet": 14471, "freeze": { "until": 1357992732 }, "description": "Payment for order No. 1234", "parameters": { "orderid": 1234 }, "transfer_id": 578842 } ], "reserve": { "until": 1357992732 }, "use_allowance": false, "suggest_allowance": false, "auto_confirm": false }
3. Confirm transaction.
When transaction status is reserved, you can confirm or revoke the transaction.
PUT https://wallet.paysera.com/rest/v1/transaction/:transaction_key/confirm
Confirmed transaction is returned on success. See get transaction information response data structure for more information.
Example request
PUT /rest/v1/transaction/pDAlAZ3z/confirm HTTP/1.1 Host: wallet.paysera.com User-Agent: Paysera WalletApi PHP library Authorization: MAC id="wkVd93h2uS", ts="1343811600", nonce="nQnNaSNyubfPErjRO55yaaEYo9YZfKHN", mac="ZQYIS0L4Uq40te+qxNXJzWHNO6Ff7qhnEDuwduFlqRI="
Example response
HTTP/1.1 200 OK Content-type: application/json;charset=utf-8
{ "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "confirmed", "type": "page", "wallet": 14471, "valid_for_payment_card_debit": false, "confirmed_at": 1355314392, "project_id": 2248, "payments": [ { "id": 2988, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "confirmed", "price": 1299, "currency": "EUR", "price_decimal": "12.99", "wallet": 14471, "confirmed_at": 1355314392, "freeze": { "until": 1357992732 }, "description": "Payment for order No. 1234", "parameters": { "orderid": 1234 } } ], "allowance": { "optional": true, "data": { "id": 784, "transaction_key": "pDAlAZ3z", "created_at": 1355314332, "status": "active", "currency": "EUR", "wallet": 14471, "confirmed_at": 1355314392, "valid": { "until": 1357992732 }, "description": "Allowance for weekly services (5 weeks)", "max_price": 1500, "max_price_decimal": "15.00", "limits": [ { "max_price": 300, "max_price_decimal": "3.00", "time": 604800 } ] } } }