Make payments to multiple beneficiaries
Paysera offers a secure API that lets you make transactions that hold multiple payments with multiple beneficiaries.
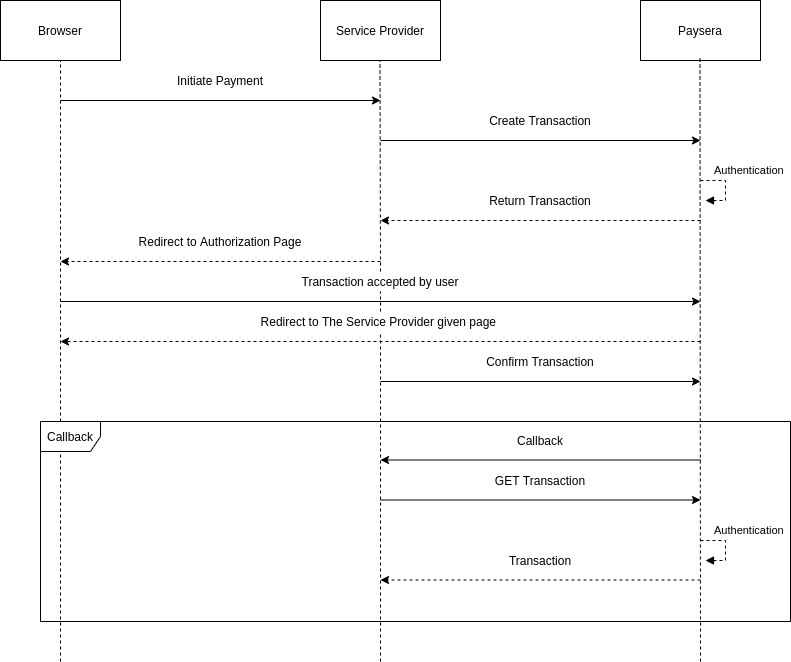
Integration
Distribute payments with a library
Create a file that will make background payment.
Create file distribute-payments.php in the paysera-wallet folder. the process is similar to
One time payment with the library, the only difference is that you
need to create multiple beneficiary
and payment
objects.
1. Create a folder.
Create a folder, name it paysera-wallet. Here, all the files related to payment creation will be stored.
2. Download the library.
Download GitHub files to the paysera-wallet folder.
3. Create a file that will create a payment.
Create file payment.php in the paysera-wallet folder. First of all you must include library and
register GatewayClient_Autoloader
as an SPL autoloader:
<php if (!class_exists('Paysera_WalletApi_Autoloader')) { require_once 'lib-wallet-php-client-master/src/Paysera/WalletApi/Autoloader.php'; } Paysera_WalletApi_Autoloader::register();
Write in your authentication data values, which you have received as Wallet credentials:
// $clientId - mac $clientId = 'wkVd93h2uS'; // $secret - mac_key $secret = 'IrdTc8uQodU7PRpLzzLTW6wqZAO6tAMU';
Create main object to use for all functionality. Using our library you may create object either for production, either for testing – Sandbox:
Production$api = new Paysera_WalletApi($clientId, $secret);Sandbox
$api = new Paysera_WalletApi($clientId, $secret, Paysera_WalletApi_Util_Router::createForSandbox());
You may create payments with detailed description for each item, more info: Payment resource.
Start new session as we will store transactionKey in $_SESSION['transactionKey']
:
session_start();
To facilitate the catching of potential exceptions code must be surrounded in try
block:
try { // Payment steps } catch (Exception $e) { echo '<pre>', $e, '</pre>'; }
Inside try block there will be logic which creates and confirms payment. First step is to create a payment object, then create a transaction with a created payment object, then authorise transaction and the last step - client (developed system) must confirm that transaction. Transaction might be confirmed automatically, after redirect to client (developed system) site or after callback. See Transaction resource for more information.
We will create two beneficiary
objects with wallet ID's
$beneficiary1 = Paysera_WalletApi_Entity_WalletIdentifier::create() ->setId($walletId1); $beneficiary2 = Paysera_WalletApi_Entity_WalletIdentifier::create() ->setId($walletId2);
To create payment object we need to create price
and item
objects which are used in
payment object. Create item with title, description, image, price and quantity:
$price = Paysera_WalletApi_Entity_Money::create() ->setAmountInCents(100) ->setCurrency('EUR') ; $item = Paysera_WalletApi_Entity_Item::create() ->setTitle('Item') ->setDescription('Item in sale') ->setImageUri('https://developers.paysera.com/bundles/evpfrontpage/img/logo.png') ->setPrice($price) ->setQuantity(2) ;
You may create and add more than one item to a payment object. In order to create payment objects and add items
we use Paysera_WalletApi_Entity_Payment
:
$payment1 = Paysera_WalletApi_Entity_Payment::create() ->addItem($item) ->setDescription('For Sale') ->setBeneficiary($beneficiary1) ; $payment2 = Paysera_WalletApi_Entity_Payment::create() ->addItem($item) ->setDescription('For Sale') ->setBeneficiary($beneficiary2) ;
Transaction object is created and values are updated using Paysera_WalletApi_Entity_Transaction. In this example we are adding two payments with a redirect link which will be used after user confirms transaction::
$transactionObj = Paysera_WalletApi_Entity_Transaction::create() ->addPayment($payment) ->setRedirectUri('http://wallet.dev.docker/payment.php') ;
After we get transaction object, we are creating the transaction:
$transactionCreated = $api->walletClient()->createTransaction($transactionObj);
User is redirected to the transaction confirmation page with a code:
header('Location:' . $api->router()->getTransactionConfirmationUri($transactionCreated->getKey()));
After user accepts transaction, client (developed system) must confirm it:
$transaction = $api->walletClient()->getTransaction($_SESSION['transactionKey']); $api->walletClient()->confirmTransaction($transaction->getKey());
Full code of distribute-payments.php:
<?php if (!class_exists('Paysera_WalletApi_Autoloader')) { require_once 'src/Paysera/WalletApi/Autoloader.php'; } Paysera_WalletApi_Autoloader::register(); $clientId = 'wkVd93h2uS'; $secret = 'IrdTc8uQodU7PRpLzzLTW6wqZAO6tAMU'; $api = new Paysera_WalletApi($clientId, $secret); session_start(); try { if (!isset($_SESSION['transactionKey'])) { $beneficiary1 = Paysera_WalletApi_Entity_WalletIdentifier::create() ->setId($walletId1); $beneficiary2 = Paysera_WalletApi_Entity_WalletIdentifier::create() ->setId($walletId2); $price = Paysera_WalletApi_Entity_Money::create() ->setAmountInCents(1) ->setCurrency('EUR') ; $item = Paysera_WalletApi_Entity_Item::create() ->setTitle('Item') ->setDescription('Item for sale') ->setImageUri('https://developers.paysera.com/bundles/evpfrontpage/img/logo.png') ->setPrice($price) ->setQuantity(1) ; $payment1 = Paysera_WalletApi_Entity_Payment::create() ->addItem($item) ->setDescription('For Sale') ->setBeneficiary($beneficiary1) ; $payment2 = Paysera_WalletApi_Entity_Payment::create() ->addItem($item) ->setDescription('For Sale') ->setBeneficiary($beneficiary2) ; $transactionObj = Paysera_WalletApi_Entity_Transaction::create() ->addPayment($payment1) ->addPayment($payment2) ->setRedirectUri('http://wallet.dev.docker/payment.php') ; $transactionCreated = $api->walletClient()->createTransaction($transactionObj); $_SESSION['transactionKey'] = $transactionCreated->getKey(); header('Location:' . $api->router()->getTransactionConfirmationUri($transactionCreated->getKey())); } if (isset($_SESSION['transactionKey'])) { $transaction = $api->walletClient()->getTransaction($_SESSION['transactionKey']); if ($transaction->getStatus() == 'reserved') { $api->walletClient()->confirmTransaction($transaction->getKey()); } unset($_SESSION['transactionKey']); } } catch (Exception $e) { echo '<pre>', $e, '</pre>'; }
Distribute payments with the specification
1. Create transaction.
Transaction groups one or more objects into one group that can be confirmed. To confirm any created object, there must be a transaction.
Moreover, transactions take part in all-or-nothing scenarios: either all of grouped payments are done
successfully, or all fail. For example, if wallet has sufficient account balance only for one of grouped
payments, user will be unable to confirm this transaction.
Transactions are also good when making shop
carts with payments to different beneficiaries - user will have to confirm something just once.
This method creates transaction that groups payment(s) and/or allowance into one item, that can be confirmed. In this example we will group already created payments. More info about transaction creation: Transaction resource.
POST https://wallet.paysera.com/rest/v1/transaction
Example request for creating payment
POST /rest/v1/transaction HTTP/1.1 Host: wallet.paysera.com Content-Type: application/json;charset=utf-8 User-Agent: Paysera WalletApi PHP library Authorization: MAC id="wkVd93h2uS", ts="1343811600", nonce="nQnNaSNyubfPErjRO55yaaEYo9YZfKHN", mac="qFRvWh+90m1qAalQyrreSTLPLP0b+6T4folfq4tJbto=", ext="body_hash=eiFuO9q%2B12FK5VIeb0BUO4tT5gaiHGsV12osAAEJWE8%3D"
{ "payments": [ { "items": [ { "title": "Item", "description": "Item for sale", "currency": "EUR", "price": "1", "quantity": 1, "parameters": { "orderid": 1111 } } ], "beneficiary": { "id": 11111111 } }, { "items": [ { "title": "Item", "description": "Item for sale", "currency": "EUR", "price": "1", "quantity": 1, "parameters": { "orderid": 2222 } } ], "beneficiary": { "id": 22222222 } } ], "redirect_uri": "http://www.example.com/somePage" }
Example response
HTTP/1.1 200 OK Content-type: application/json;charset=utf-8
{ "transaction_key": "pDAlAZ3z", "created_at": 1700746829, "status": "new", "valid_for_payment_card_debit": true, "reserve": { "for": 2592000 }, "use_allowance": true, "suggest_allowance": false, "auto_confirm": false, "redirect_uri": "http://wallet.dev.docker/payment.php", "project_id": 111111111, "manager_id": 111111111, "payments": [ { "id": 111111111, "transaction_key": "pDAlAZ3z", "created_at": 1700746829, "status": "new", "cancelable": false, "price": 1, "currency": "EUR", "price_decimal": "0.01", "freeze": { "for": 0 }, "freeze_for": 0, "description": "Item", "items": [ { "title": "Item", "description": "Item for sale", "price": 1, "currency": "EUR", "price_decimal": "0.01", "quantity": 1, "parameters": { "orderid": 1111 }, "total_price": 1, "total_price_decimal": "0.01" } ], "beneficiary": { "id": 111111111 } }, { "id": 111111111, "transaction_key": "pDAlAZ3z", "created_at": 1700746829, "status": "new", "cancelable": false, "price": 1, "currency": "EUR", "price_decimal": "0.01", "freeze": { "for": 0 }, "freeze_for": 0, "description": "Item", "items": [ { "title": "Item", "description": "Item for sale", "price": 1, "currency": "EUR", "price_decimal": "0.01", "quantity": 1, "parameters": { "orderid": 2222 }, "total_price": 1, "total_price_decimal": "0.01" } ], "beneficiary": { "id": 222222222 } } ], "inquiries": [] }
transaction_key
of all objects in the transaction are the same and equals to that of
transaction itself
2. Authorising transaction.
After creating transaction, it has to be authorised by a user and confirmed by the client (developed system) to take effect. Authorising (reserving funds) can be accomplished by redirecting user to transaction confirmation page in Paysera system. This way user leaves your site and comes back only after confirming or canceling the request. You should provide redirect_uri when creating the transaction if you are using this confirmation way. If not provided, user will see notice and will not be redirected anywhere after confirming or rejecting the transaction.
In English
https://www.paysera.com/frontend/en/wallet/confirm/:transaction_key
In Lithuanian
https://www.paysera.com/frontend/wallet/confirm/:transaction_key
In Russian
https://www.paysera.com/frontend/ru/wallet/confirm/:transaction_key
More info about authorising transaction: Authorising transactions (reserving funds).
3. Confirm transaction.
When transaction status is reserved, you can confirm or revoke the transaction.
PUT https://wallet.paysera.com/rest/v1/transaction/:transaction_key/confirm
Confirmed transaction is returned on success. See get transaction information response data structure for more information.
Example request
PUT /rest/v1/transaction/pDAlAZ3z/confirm HTTP/1.1 Host: wallet.paysera.com User-Agent: Paysera WalletApi PHP library Authorization: MAC id="wkVd93h2uS", ts="1343811600", nonce="nQnNaSNyubfPErjRO55yaaEYo9YZfKHN", mac="ZQYIS0L4Uq40te+qxNXJzWHNO6Ff7qhnEDuwduFlqRI="
Example response
HTTP/1.1 200 OK Content-type: application/json;charset=utf-8
{ "transaction_key": "pDAlAZ3z", "created_at": 1701257481, "status": "confirmed", "type": "page", "wallet": 111111111, "valid_for_payment_card_debit": false, "reserve": { "until": 1703849539, "for": 2592000 }, "confirmed_at": 1701257577, "auto_confirm": false, "redirect_uri": "http://www.example.com/somePage", "project_id": 111111111, "manager_id": 11111111, "payments": [ { "id": 386892298, "transaction_key": "pDAlAZ3z", "created_at": 1701257481, "status": "done", "cancelable": false, "price": 1, "currency": "EUR", "price_decimal": "0.01", "wallet": 111111111, "confirmed_at": 1701257577, "freeze": { "for": 0 }, "freeze_for": 0, "description": "Item", "items": [ { "title": "Item", "description": "Item for sale", "price": 1, "currency": "EUR", "price_decimal": "0.01", "quantity": 1, "parameters": { "orderid": 1111 }, "total_price": 1, "total_price_decimal": "0.01" } ], "transfer_id": 665719449, "beneficiary": { "id": 111111111 } }, { "id": 386892299, "transaction_key": "pDAlAZ3z", "created_at": 1701257481, "status": "done", "cancelable": false, "price": 1, "currency": "EUR", "price_decimal": "0.01", "wallet": 222222222, "confirmed_at": 1701257577, "freeze": { "for": 0 }, "freeze_for": 0, "description": "Item", "items": [ { "title": "Item", "description": "Item for sale", "price": 1, "currency": "EUR", "price_decimal": "0.01", "quantity": 1, "parameters": { "orderid": 2222 }, "total_price": 1, "total_price_decimal": "0.01" } ], "transfer_id": 665719449, "beneficiary": { "id": 222222222 } } ], "inquiries": [] }